文章请参考:
http://caterpillar.onlyfun.net/Gossip/DesignPattern/index.html
-> 表示类的继承
1.factory
f -> concreateF
p->concreatP
f.createP
concreateF.createP(return new concreateP)
2.abstract factory:
af
af->cf1
af->cf2
apa->pa1
apa->pa2
apb->pb1
apb->pb2
cf1.createA(return new pa1)
cf1.createB(return new pb1)
cf2.createA(return new pa2)
cf2.createB(return new pb2)
3.singleton
A
最简单的例子:
这句话解释的最好:
对象分析和设计中其实基于两个原则:
a.一个类只有一个引起它变化的原因
b.使用组合而不是继承来实现功能需求
实现方式:
就是当类有两个以上的强烈变化的原因的时候,使用birdge而非继承,避免类的数量过于膨胀。
2. Adapter 模式
就我理解是把一个interface 转化为另外一个interface,一定是interface而不是类。
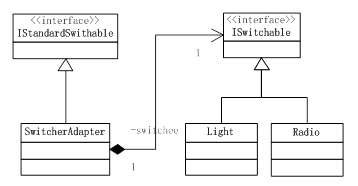
Adapter,表示遇到的问题是接口不匹配。
Proxy,表示遇到的问题是主体逻辑与附加逻辑(持久化、网络传输等)纠缠。
这两句话解释了很多问题。
3. Decorator 模式
the same as price. by this way we can add functions or make modification without hieritage.

4. composite 模式:
interface P: play()
P->Frame
P->PList:list
add Frame in Plist:list
and call Frame.play() in Plist.play for each list element.
5. flyweight 模式:
把也许会重复生成的小object 放在一个静态hash表里(可以通过factory模式来实现这个hash表)。create一个小object时,先看这个hash表里有没有,没有就生成一个并在hash表里添加这个object的指针,如果有就不重新生成,直接从hash表里取出,前提是这个object并不会被其他的程序所更改。
6.facade模式
如果你的函数中需要调用很多库的类,那么你的函数就会与库有很高的耦合度,如果库有变化,那么你就需要改写每一处使用这些库的地方,facade模式就是抽象出一个service,让service提供一些函数来实现对库的操作或者是组合内容,这样你的函数中就只需要调用service的函数,好处是库变化是,你只需要更改你的service,而不用更改你每一处用到库的地方。不好是你又加了一层复杂度,有时候会变得更加难以理解。
7. proxy模式
比如这个:

在原始逻辑上添加一层而已。
这是後通过构造一个统一的基础虚类,然后把不同的操作实作为衍生类,然后通过他们之间的调用顺序来实现不同的执行顺序,避免了大量的if then,语义上看起来也比较清晰

这个uml图就很好解释了这个关系,receiver不一定要封装在command中,也可以注入
strategy就是:
这个图说的很明白,就好比需要一个中间人来处理一群共事的同时之间的关系,使得团队各个成员的工作彼此独立,交互更有弹性。(比如更换成员,比如更改联系方式)
http://caterpillar.onlyfun.net/Gossip/DesignPattern/index.html
-> 表示类的继承
1.factory
f -> concreateF
p->concreatP
f.createP
concreateF.createP(return new concreateP)
2.abstract factory:
af
af->cf1
af->cf2
apa->pa1
apa->pa2
apb->pb1
apb->pb2
cf1.createA(return new pa1)
cf1.createB(return new pb1)
cf2.createA(return new pa2)
cf2.createB(return new pb2)
3.singleton
A
A::instance(){ return this.instance or this.instance=new A}使用:
a=A::instance()4.builder
最简单的例子:
1: //Client同时充当了Director的角色
2: StringBuilder builder = new StringBuilder();
3: builder.Append("happyhippy");
4: builder.Append(".cnblogs");
5: builder.Append(".com");
6: //返回string对象:happyhippy.cnblogs.com
7: builder.ToString();
builder模式主要是有个build part的过程,这个过程叫director,它build的参数,顺序不同都是不同的结果,而abstract factory没有这个过程这句话解释的最好:
客户端调用:director负责发布命令,builder才负责真正的生产,所以向builder要产品而不是director
//调用 public class Client { public static void main(String[] args) { Builder builder = new ConcreteBuilder(); Director director = new Director( builder ); director.construct(); //builder负责真正的生产 Product product = builder.getResult(); //向builder要产品而不是向director要产品 }
是想builder要产品,更换不同的director, builder能够得到不同的产品
1、需要生成的产品对象有复杂的内部结构。
2、需要生成的产品对象的属性相互依赖,建造者模式可以强迫生成顺序。
2、需要生成的产品对象的属性相互依赖,建造者模式可以强迫生成顺序。
3、当复杂对象的创建应该独立于该对象的组成部分和装配方式时
但是有个问题是这个builder就必须以传址的方式传递给director。
5. prototype
就是
P::clone
p1=p.clone();
复制本身。
结构型模式
1.bridge模式对象分析和设计中其实基于两个原则:
a.一个类只有一个引起它变化的原因
b.使用组合而不是继承来实现功能需求
实现方式:
就是当类有两个以上的强烈变化的原因的时候,使用birdge而非继承,避免类的数量过于膨胀。
AbstractRoad AbstractRoad->SpeedWay AbstractRoad->Street AbstractP AbstractP->Man AbstractP->Woman AbstractV AbstractV::run() AbstractV::road AbstractV::P AbstractV->Car AbstractV->Bus car.p=Man car.road=SpeedWay car.run() or b us.p=woman bus.road=street bus.run()这是一个简单的例子
2. Adapter 模式
就我理解是把一个interface 转化为另外一个interface,一定是interface而不是类。
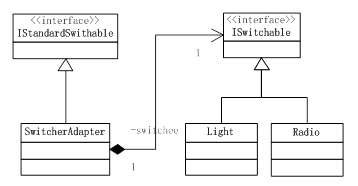
Adapter,表示遇到的问题是接口不匹配。
Proxy,表示遇到的问题是主体逻辑与附加逻辑(持久化、网络传输等)纠缠。
这两句话解释了很多问题。
3. Decorator 模式
interface Meal {getContent(), price()} Meal->FriedChiken Meal->FiredHamburger Meal-> abstract SideDish SideDish->DishOne SideDish->DishTwo Meal meal=DishOne(FriedChicken); meal.getContent() meal.price()in dishone, the getContent call the getcontent of friedChicken and combine the result.
the same as price. by this way we can add functions or make modification without hieritage.

4. composite 模式:
interface P: play()
P->Frame
P->PList:list
add Frame in Plist:list
and call Frame.play() in Plist.play for each list element.
5. flyweight 模式:
把也许会重复生成的小object 放在一个静态hash表里(可以通过factory模式来实现这个hash表)。create一个小object时,先看这个hash表里有没有,没有就生成一个并在hash表里添加这个object的指针,如果有就不重新生成,直接从hash表里取出,前提是这个object并不会被其他的程序所更改。
6.facade模式
如果你的函数中需要调用很多库的类,那么你的函数就会与库有很高的耦合度,如果库有变化,那么你就需要改写每一处使用这些库的地方,facade模式就是抽象出一个service,让service提供一些函数来实现对库的操作或者是组合内容,这样你的函数中就只需要调用service的函数,好处是库变化是,你只需要更改你的service,而不用更改你每一处用到库的地方。不好是你又加了一层复杂度,有时候会变得更加难以理解。
7. proxy模式
比如这个:

在原始逻辑上添加一层而已。
行为模式
1.Template模式
将父类作为骨架,将实作的实现放到子类来填充,
2. Chaine of responsiblity 模式
具体用例请参见文章开始的引文,我的理解是这样的:
如果你有一个procedure,比如这种:
if a then do a else if b then do b else if .... endif这种情况下,如果你的处理流程改了,需要增加或者更改顺序,(比如a ,b ...上有语义上的包含关系,更改顺序会使得结果截然不同),那么你都需要重新更改这段程序,而且如果这种关系比较复杂,那么更改的时候会比较痛苦。
这是後通过构造一个统一的基础虚类,然后把不同的操作实作为衍生类,然后通过他们之间的调用顺序来实现不同的执行顺序,避免了大量的if then,语义上看起来也比较清晰
h=new a(new b(new c())); h.handle()就解决了,而且也把不同的处理流程完全分开了,使得程序的耦合性降低。
3. command 模式
这个模式很常见,我自己都常常在用,比如说在spoop中的那个controller class, command 传入以后, 调用CommandAction,将receiver放在传回的值当中或者用JsonResponse中,
这个uml图就很好解释了这个关系,receiver不一定要封装在command中,也可以注入
4. strategy 模式
这个模式的核心思想和template很像
strategy就是:
Server server = new Server(9999, new EchoService()); server.start();template是:
GuessGame game = new ConsoleGame(); game.go();strategy 是传入不同的组件来实现不同的服务,template是用不同的子类来实现通过父类定义好的模板。
5. observer 模式
这个就是消息分发机制的抽象,当subject 管理一个obeserver列表,当subject的状态改变时,通知列表里的obersever,obersever此时执行update().Mediator 模式

这个图说的很明白,就好比需要一个中间人来处理一群共事的同时之间的关系,使得团队各个成员的工作彼此独立,交互更有弹性。(比如更换成员,比如更改联系方式)
评论
发表评论